Introduction
Brief Introduction to Graph Algorithms
Graph algorithms are a cornerstone of computer science, used to solve a myriad of problems in various fields such as social networks, biology, logistics, and computer networks. Graphs consist of nodes (vertices) connected by edges and can represent anything from a network of roads to the connections in a social network. Traversing these graphs efficiently is crucial, and this is where graph algorithms come into play.
Importance of DFS and BFS in Computer Science
Two of the most fundamental graph traversal algorithms are Depth-First Search (DFS) and Breadth-First Search (BFS). These algorithms are essential for exploring the nodes and edges of a graph systematically. Understanding DFS and BFS is crucial for tackling problems related to connectivity, pathfinding, and network flow. They form the foundation for more complex algorithms and applications, making them indispensable tools for computer scientists and engineers.
Overview of What the Article Will Cover
This article will delve into the intricacies of DFS and BFS, providing a detailed explanation of each algorithm, their working mechanisms, and practical applications. We will compare the two algorithms, highlighting their differences and use cases. Additionally, examples with simple graphs will illustrate how each algorithm traverses a graph, making the concepts clear and easy to understand.
What is Depth-First Search (DFS)?
Definition and Basic Explanation
Depth-First Search (DFS) is a graph traversal algorithm that explores as far down a branch as possible before backtracking. It starts at a chosen node (often called the root) and moves forward along a path until it can go no further, at which point it backtracks to explore other paths.
How DFS Works (Step-by-Step Process)
Start at the Root Node: Begin at the root node or an arbitrary starting node in the graph.
Visit the Node: Mark the current node as visited.
Explore Adjacent Nodes: Move to an adjacent, unvisited node.
Repeat: Repeat the process for each unvisited node, going deeper into the graph.
Backtrack: If no adjacent unvisited nodes are available, backtrack to the previous node and continue the process.
Complete: Continue until all nodes have been visited.
Example of DFS Traversal with a Simple Graph
Consider a graph with nodes A, B, C, and D, and edges connecting A-B, A-C, and B-D. Starting at node A, a DFS traversal might visit the nodes in the order A, B, D, and then C.
Key Points
Approach: Depth-first exploration, diving deep into each branch before backtracking.
Data Structure Used: Typically uses a stack, which can be implemented using recursion.
Use Cases and Applications:
Topological sorting
Finding connected components
Solving puzzles and games (e.g., mazes)
Time and Space Complexity of DFS
Time Complexity: O(V + E), where V represents the number of vertices and E represents the number of edges in the graph. This indicates that DFS visits every vertex and traverses every edge in the graph.
Space Complexity: O(V), which arises from the stack used to keep track of vertices for backtracking, either through explicit stack data structures or recursive function calls.
What is Breadth-First Search (BFS)?
Definition and Basic Explanation
Breadth-First Search (BFS) is a graph traversal algorithm that systematically explores the nodes at the current depth level before progressing to the next level. Starting from a designated root node, BFS visits all its neighbors first, before moving on to the neighbors of those neighbors, ensuring that nodes are explored in increasing order of their distance from the root.
How BFS Works (Step-by-Step Process)
Start at the Root Node: Begin at the root node or an arbitrary starting node in the graph.
Visit the Node: Mark the current node as visited.
Enqueue Adjacent Nodes: Add all unvisited adjacent nodes to a queue.
Dequeue and Visit: Remove the next node from the queue and visit it.
Repeat: Repeat the process for each node, exploring nodes level by level.
Complete: Continue until the queue is empty and all nodes have been visited.
Example of BFS Traversal with a Simple Graph
Consider a graph with nodes A, B, C, and D, and edges connecting A-B, A-C, and B-D. Starting at node A, a BFS traversal might visit the nodes in the order A, B, C, and then D.
Key Points
Approach: Level-by-level exploration, visiting all nodes at the current depth before moving deeper.
Data Structure Used: Typically uses a queue to manage the nodes to be explored.
Use Cases and Applications:
Finding the shortest path in unweighted graphs
Level-order traversal of trees
Network broadcasting
Time and Space Complexity:
Time Complexity: O(V + E), where V represents the number of vertices and E represents the number of edges in the graph.
Space Complexity: O(V) due to the queue used to store nodes.
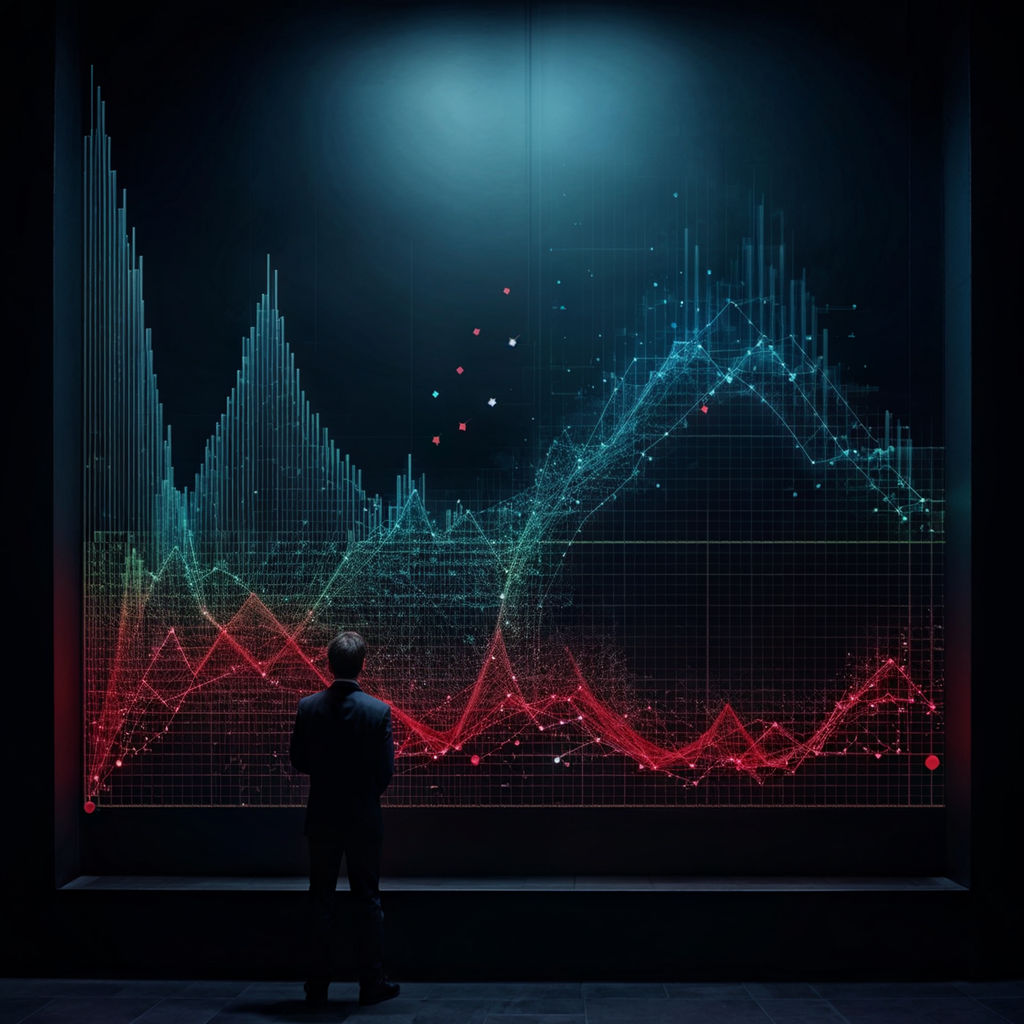
DFS vs. BFS: A Comparative Analysis
Side-by-Side Comparison Table
Feature | DFS (Depth-First Search) | BFS (Breadth-First Search) |
Approach | Depth-first exploration | Level-by-level exploration |
Data Structure | Stack (can use recursion) | Queue |
Order of Visiting Nodes | As deep as possible before backtracking | All neighbors at the present depth first |
Use Cases | Topological sorting, finding connected components, solving puzzles | Shortest path in unweighted graphs, level-order traversal, network broadcasting |
Time Complexity | O(V + E) | O(V + E) |
Space Complexity | O(V) | O(V) |
When to Use DFS vs. BFS
- DFS: Choose DFS when you need to explore as far as possible down one path before backtracking. It’s useful for tasks that involve backtracking, such as puzzles or games, and for problems where you need to visit all nodes of a graph, such as topological sorting or finding connected components.
BFS: Opt for BFS when you need to explore nodes level by level. BFS is ideal for finding the shortest path in unweighted graphs and for problems that require visiting nodes in increasing order of their distance from the source, such as network broadcasting or level-order traversal of trees.
Real-world Applications of DFS
Examples of Where DFS is Used in Real-world Problems
Topological Sorting: DFS can be leveraged to execute a topological sort on a Directed Acyclic Graph (DAG), ensuring a linear ordering of vertices that respects the directed edges’ dependencies. This is useful in scenarios where tasks must be ordered based on dependencies, such as scheduling jobs or compiling code.
Finding Connected Components: In undirected graphs, DFS helps identify connected components, which is useful in network analysis to find clusters or communities.
Solving Puzzles (e.g., Mazes): DFS explores all possible paths in a maze, allowing it to find a solution if one exists. It’s also used in solving problems like the N-Queens problem or Sudoku.
Real-world Applications of BFS
Examples of Where BFS is Used in Real-world Problems
Shortest Path in Unweighted Graphs: BFS is used to find the shortest path between two nodes in an unweighted graph. This is applicable in routing and navigation systems.
Level-order Traversal of Trees: BFS can traverse a tree level by level, which is useful in various applications such as printing a tree in level order or performing level-order operations on data structures.
Network Broadcasting: BFS ensures that all nodes receive a broadcast message in the shortest number of steps. This is important in network communication protocols.
Conclusion
Summary of Key Points
DFS and BFS are fundamental graph traversal algorithms, each with distinct approaches and use cases.
DFS is depth-first, using a stack and is ideal for tasks requiring thorough exploration of each path.
BFS is breadth-first, using a queue and is suitable for finding the shortest path in unweighted graphs and level-order traversals.
Importance of Understanding Both DFS and BFS
Understanding both DFS and BFS is crucial for solving a wide range of problems in computer science. These algorithms form the foundation for more advanced techniques and applications in various domains.
Encouragement to Practice Both Algorithms
To master these algorithms, practice implementing them in different scenarios and explore their applications in real-world problems. Experimenting with DFS and BFS will enhance your problem-solving skills and deepen your understanding of graph theory.